Richardson-Lucy-Deconvolution on OpenCL-compatible GPUs#
Richardson-Lucy-Deconvolution is a common and yet basic algorithm for image deconvolution in microscopy. In this notebook we will use a GPU-accelerated version of it that is implemented in the napari-plugin RedLionFish. Hence, you can use the same algorithm from the graphical user interface in napari.
from skimage.io import imread
from pyclesperanto_prototype import imshow
import RedLionfishDeconv as rl
import matplotlib.pyplot as plt
We will load an image showing fluorescent intensity along lines. This 3D image was taken with a confocal microscope.
image = imread('../../data/DeconvolutionSampleVerticalGrid1AU-crop.tif')
image.shape
(21, 150, 150)
imshow(image, colorbar=True)
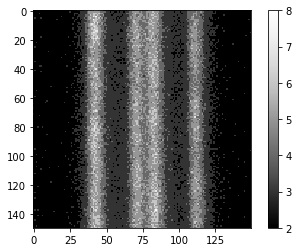
The following PSF image was extracted from images taken with the same microscope using the procedure explained before.
psf = imread('../../data/psf.tif')
imshow(psf, colorbar=True)
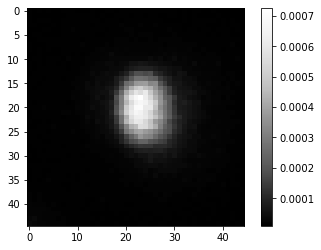
We can now deconvolve the image using RedLionFish’s Richardson-Lucy-Deconvolution algorithm. We should specify that the algorith shall be executed on the gpu
.
iterations = 50
deconvolved = rl.doRLDeconvolutionFromNpArrays(image,
psf,
niter=iterations,
method='gpu',
resAsUint8=False )
imshow(deconvolved)
ERROR:root:Failed to setup Reikna with OpenCL.
ERROR:root:No module named 'reikna'
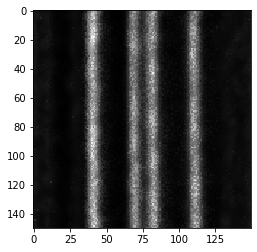
To visualize more precisely how the original image and the deconvolved version differ, we can plot the intensity along a line from the left to the right. We retrieve these numbers from a maximum intensity projection along Z.
max_intensity_image = image.max(axis=0)
max_intensity_image.shape
(150, 150)
max_intensity_deconvolved = deconvolved.max(axis=0)
max_intensity_deconvolved.shape
(150, 150)
plt.plot(max_intensity_image[80])
plt.plot(max_intensity_deconvolved[80])
plt.show()
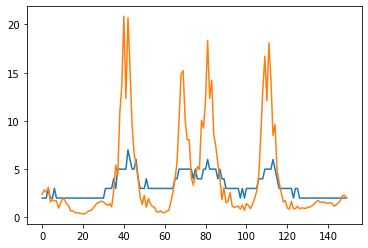
As you can see, the intensity range has change through deconvolution. This depends on the algorithm and implementation. Whenever applying deconvolution, consider checking if the total intensity in the original image and the deconvolved image are within the same range:
image.min(), image.max()
(1, 8)
deconvolved.min(), deconvolved.max()
(0.0, 28.122286)