Object segmentation on OpenCL-compatible GPUs#
APOC is based on pyclesperanto and scikit-learn. For object segmentation, it uses a pixel classifier and connected components labeling.
Let’s start with loading an example image and some ground truth:
from skimage.io import imread, imsave
import pyclesperanto_prototype as cle
import numpy as np
import apoc
image = imread('../../data/blobs.tif')
cle.imshow(image)
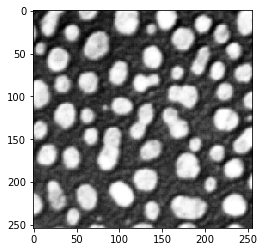
manual_annotations = imread('../../data/blobs_annotations.tif')
cle.imshow(manual_annotations, labels=True)
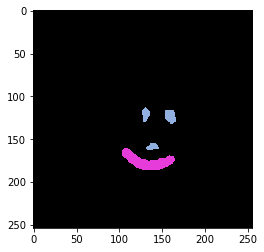
Training#
We now train a ObjectSegmenter, which is under the hood a scikit-learn RandomForestClassifier. After training, the classifier will be converted to clij-compatible OpenCL code and save to disk under a given filename.
# define features
features = apoc.PredefinedFeatureSet.medium_quick.value
# this is where the model will be saved
cl_filename = '../../data/blobs_object_segmenter.cl'
apoc.erase_classifier(cl_filename)
clf = apoc.ObjectSegmenter(opencl_filename=cl_filename, positive_class_identifier=2)
clf.train(features, manual_annotations, image)
Prediction / segmentation#
The classifier can then be used to classify all pixels in the given image. Starting point is again, the feature stack. Thus, the user must make sure that the same features are used for training and for prediction. Prediction can be done on the CPU using the original scikit-learn code and on the GPU using the generated OpenCL-code. OCLRFC works well if both result images look identical.
segmentation_result = clf.predict(features=features, image=image)
cle.imshow(segmentation_result, labels=True)
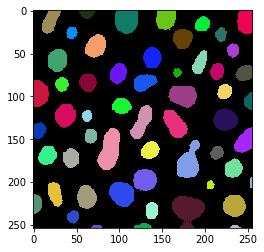
Segmentation from a loaded segmenter#
clf = apoc.ObjectSegmenter(opencl_filename=cl_filename)
segmentation_result = clf.predict(image=image)
cle.imshow(segmentation_result, labels=True)
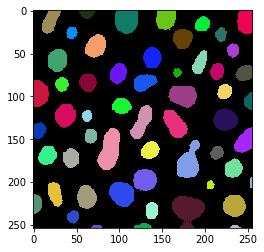