(image_file_formats:aicsimagio=)
Reading files with AICSImageIO#
The AICSImageIO library aims streamlining reading microscopy image data.
To install the library, you need to call this command.
pip install aicsimageio
In case specific proprietary file formats should be read, additional software must be installed. Check the documentation for details.
from aicsimageio import AICSImage
from skimage.io import imshow
First, we create an AICSImage
object to see if it understands our file format. In the following example, we read an OME Tif file that was saved with ImageJ before.
As example we are using here an image shared by Célia Baroux et al(University of Zurich) that was resaved for demonstration purposes.
aics_image = AICSImage("../../data/EM_C_6_c0.ome.tif")
aics_image
Attempted file (C:/structure/code/BioImageAnalysisNotebooks/data/EM_C_6_c0.ome.tif) load with reader: aicsimageio.readers.bfio_reader.OmeTiledTiffReader failed with error: No module named 'bfio'
C:\Users\haase\mambaforge\envs\bio39\lib\site-packages\ome_types\_convenience.py:105: FutureWarning: The default XML parser will be changing from 'xmlschema' to 'lxml' in version 0.4.0. To silence this warning, please provide the `parser` argument, specifying either 'lxml' (to opt into the new behavior), or'xmlschema' (to retain the old behavior).
d = to_dict(os.fspath(xml), parser=parser, validate=validate)
<AICSImage [Reader: OmeTiffReader, Image-is-in-Memory: False]>
This object can already give us basic information such as image size/shape, dimensions and dimension names and order.
aics_image.shape
(1, 1, 256, 256, 256)
aics_image.dims
<Dimensions [T: 1, C: 1, Z: 256, Y: 256, X: 256]>
aics_image.dims.order
'TCZYX'
From this object, we can also retrieve pixels as numpy arrays.
np_image = aics_image.get_image_data("ZYX", T=0)
np_image.shape
(256, 256, 256)
imshow(np_image[128])
<matplotlib.image.AxesImage at 0x158c9b60ac0>
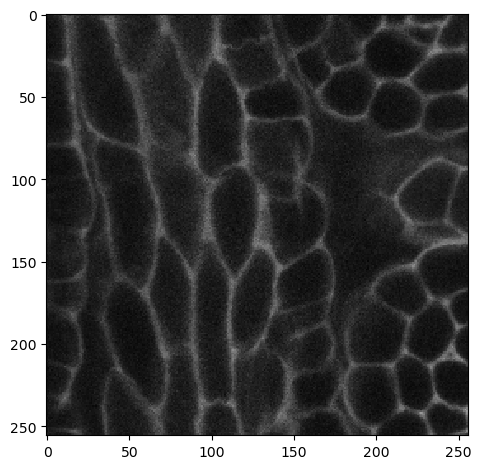
Reading meta data#
When working with microscopy image data, it is important to be aware of meta data, for example the voxel size. In order to do volume measurements in proper physical units, we need to know how large a voxel is in X, Y and Z.
aics_image.physical_pixel_sizes
PhysicalPixelSizes(Z=0.16784672897196262, Y=0.16776018346253663, X=0.16776018346253663)
And one can define a helper function for reading the voxel size in Z/Y/X format.
def get_voxel_size_from_aics_image(aics_image):
return (aics_image.physical_pixel_sizes.Z,
aics_image.physical_pixel_sizes.Y,
aics_image.physical_pixel_sizes.X)
get_voxel_size_from_aics_image(aics_image)
(0.16784672897196262, 0.16776018346253663, 0.16776018346253663)
Reading CZI files#
In case additionally the aicspylibczi
library is installed one can also open CZI files using AICSImageIO.
czi_image = AICSImage("../../data/PupalWing.czi")
czi_image.shape
(1, 1, 80, 520, 692)
np_czi_image = czi_image.get_image_data("ZYX", T=0)
np_czi_image.shape
(80, 520, 692)
get_voxel_size_from_aics_image(czi_image)
(1.0, 0.20476190476190476, 0.20476190476190476)
Reading LIF files#
In case additionally the readlif
library is installed, one can also read LIF images using AICSImageIO.
lif_image = AICSImage("../../data/y293-Gal4_vmat-GFP-f01.lif")
lif_image.shape
(1, 2, 86, 500, 616)
np_lif_image = lif_image.get_image_data("ZYX", T=0)
np_lif_image.shape
(86, 500, 616)
get_voxel_size_from_aics_image(lif_image)
(1.0070810588235295, 0.46827875751503006, 0.46827869918699183)