Merging annotated labels#
In this notebook we demonstrate how a label-image can be post-processed by annotating labels that should be merged.
import apoc
from skimage.io import imread, imshow, imsave
import pyclesperanto_prototype as cle
import numpy as np
Our starting point is an oversegmented (synthetic) label image.
oversegmented = cle.asarray(imread('../../data/syntetic_cells.tif')).astype(np.uint32)
oversegmented
|
cle._ image
|
Furthermore, we need an annotation where pixel-intensity = 1 implies that labels should be merged.
annotation = cle.asarray(imread('../../data/syntetic_cells_merge_annotation.tif')).astype(np.uint32)
# binarize the image
annotation = annotation == 1
annotation
|
cle._ image
|
For visualization purposes, we overlay both.
cle.imshow(oversegmented, labels=True, continue_drawing=True)
cle.imshow(annotation, alpha=0.5)
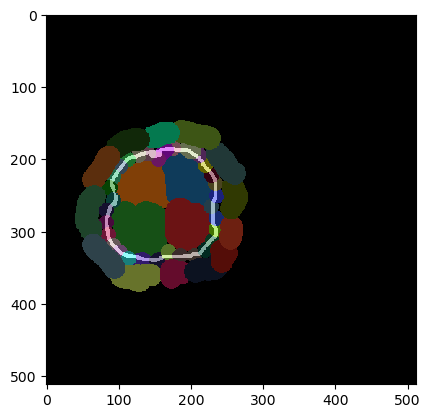
We can now merge all cells whose borders are annotated.
result = cle.merge_annotated_touching_labels(oversegmented, annotation)
result
|
cle._ image
|
How does it work?#
Under the hood, there is a function for generating a touch-matrix from the label image and the annotation and a function for merging labels according to a touch-matrix.
should_touch_matrix = cle.generate_should_touch_matrix(oversegmented, annotation)
should_touch_matrix
|
cle._ image
|
result = cle.merge_labels_according_to_touch_matrix(oversegmented, should_touch_matrix)
result
|
cle._ image
|