Generating Magnetic Resonance images using DALL-E#
In this notebook we will demonstrate how to ask openAI’s DALL-E model to generate medial images (for fun).
Read more:
import openai
from skimage.io import imread, imshow
from numpy import random
from matplotlib import pyplot as plt
def prompt_image(message:str, width:int=1024, height:int=1024, model='dall-e-3'):
client = openai.OpenAI()
response = client.images.generate(
prompt=message,
model=model,
n=1,
size=f"{width}x{height}"
)
image_url = response.data[0].url
image = imread(image_url)
return image
As a real example of an orange slice imaged with MR, we use the example dataset “Credit: Mandarin orange, axial view, MRI.” is licensed (CC-BY 4.0) by Alexandr Khrapichev, University of Oxford
images = [imread('../../data/mri_fruit_sxm89b3x.jpg')[3063:4087,1024:2048,0]]
mri_prompt = """
A single, high resolution, black-white image of
a realistically looking orange fruit slice
imaged with T2-weighted magnetic resonance imaging (MRI).
"""
for _ in range(3):
images.append(prompt_image(mri_prompt))
random.shuffle(images)
fix, ax = plt.subplots(1,len(images), figsize=(15,15))
for i, image in enumerate(images):
ax[i].imshow(image, cmap='Greys_r')
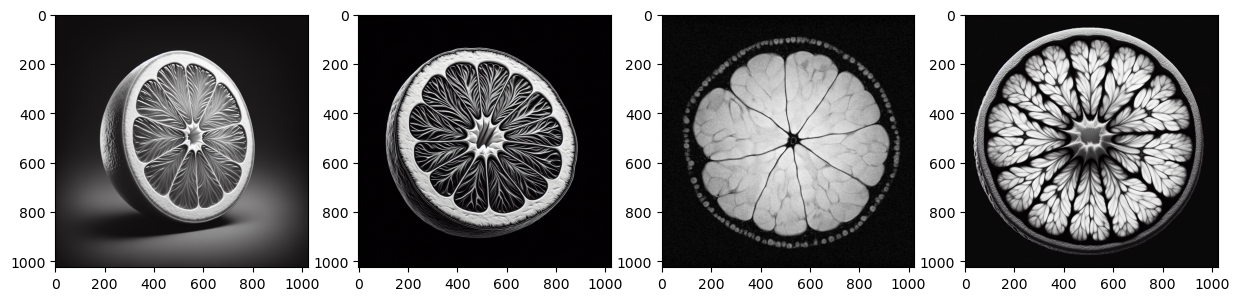
Exercise#
There is another example data set available. Crop out the star fruit from that image and repeat the experiment: Write a prompt that generates images looking similar.
The example dataset “Collage of mixed fruits and vegetables, MRI.” is licensed (CC-BY 4.0) by Alexandr Khrapichev, University of Oxford
image2 = imread('../../data/mri_fruit_bvtnk4mm.jpg')
imshow(image2)
<matplotlib.image.AxesImage at 0x10a35ff4ac0>
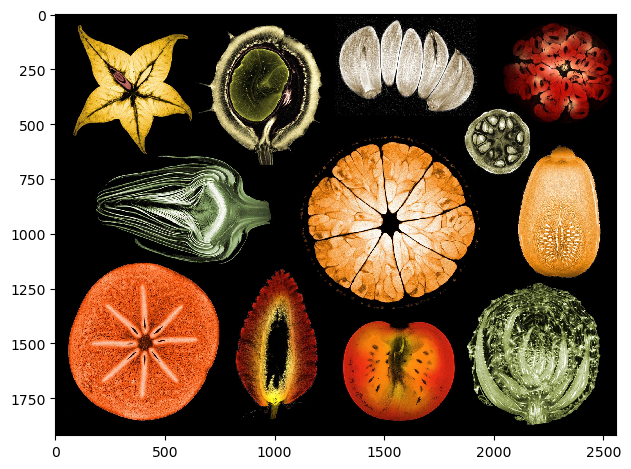