Binary Image Skeletonization#
This notebook demonstrates basic binary image skeletonization using Python libraries.
Introduction#
Skeletonization reduces binary objects in an image to their essential lines, preserving the structure and connectivity, allowing for further analysis of the shapes and structures.
# Import necessary libraries
import matplotlib.pyplot as plt
from skimage import data, filters, morphology, color
Load and preprocess the image#
We’ll use a sample image from skimage.data
and convert it to binary form for demonstration.
# Load sample image
image = data.horse() # Sample binary image
# Apply threshold to convert to binary image
binary_image = image < filters.threshold_otsu(image)
# Plot original and binary images
fig, ax = plt.subplots(1, 2, figsize=(8, 4))
ax[0].imshow(image, cmap='gray')
ax[0].set_title('Original Image')
ax[1].imshow(binary_image, cmap='gray')
ax[1].set_title('Binary Image')
plt.show()
<__array_function__ internals>:180: RuntimeWarning: Converting input from bool to <class 'numpy.uint8'> for compatibility.
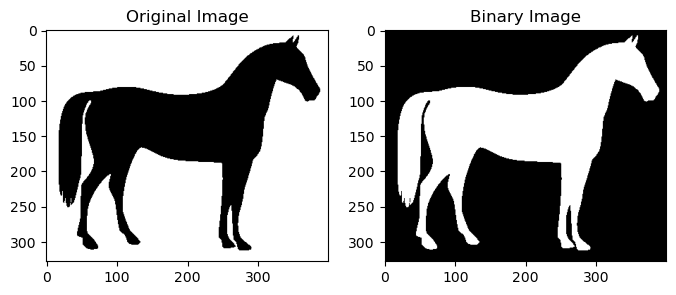
Perform Skeletonization#
We’ll use the skeletonize
function from skimage.morphology
to perform skeletonization on the binary image.
# Perform skeletonization
skeleton = morphology.skeletonize(binary_image)
# Plot skeletonized image
plt.figure(figsize=(4, 4))
plt.imshow(skeleton, cmap='gray')
plt.title('Skeletonized Image')
plt.show()
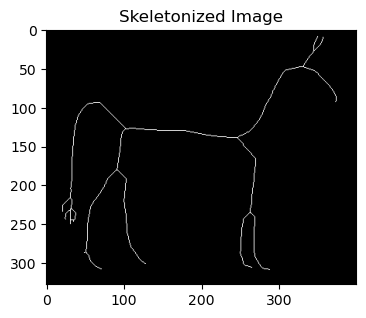