Accessing image files in the owncloud#
NOTE: This notebook has been moded to the section “remote files”
This notebook we will demonstrate how to download files from an owncloud server, how to process it locally and upload the results back to the server.
Depending on which cloud we use, we may have to install different libaries.
Owncloud installation
pip install pyocclient
Nextcloud installation
pip install pyncclient
from skimage.io import imread, imshow, imsave
from napari_segment_blobs_and_things_with_membranes import voronoi_otsu_labeling
import ipywidgets as widgets
import owncloud
# import nextcloud_client as owncloud
Login#
After executing the next cell locally, you will see three input fields where you can enter the server url, username and password.
server_widget = widgets.Text(value='https://sharing.biotec.tu-dresden.de', description='Server')
username_widget = widgets.Text(description='Username:')
password_widget = widgets.Password(description='Password')
widgets.VBox([server_widget, username_widget, password_widget])
Do NOT hit Shift-Enter after entering username and password
if username_widget.value == '':
raise RuntimeError('Enter username and password above')
oc = owncloud.Client(server_widget.value)
oc.login(username_widget.value, password_widget.value)
A typical error message is 401: Access denied. Check your username and password.
Browsing the remote server#
To see if the connection was successful, we can just list the files in a given folder.
# enter a folder on the owncloud drive that exists. '/' is the root folder.
remote_folder = "/data/"
for f in oc.list(remote_folder):
print (f.path)
/data/blobs.tif
/data/blobs_labels.tif
/data/testfolder/
/data/zfish_nucl_env.tif
import tempfile
temp_folder = tempfile.TemporaryDirectory(prefix="napari-owncloud")
import os
temp_dir = temp_folder.name.replace("\\", "/") + remote_folder
os.path.isdir(temp_dir)
True
os.mkdir(temp_dir)
---------------------------------------------------------------------------
FileExistsError Traceback (most recent call last)
Cell In [12], line 1
----> 1 os.mkdir(temp_dir)
FileExistsError: [WinError 183] Cannot create a file when that file already exists: 'C:/Users/haase/AppData/Local/Temp/napari-owncloudc0epk0iu/data/'
Retrieving a file#
We can download a file to a local directory.
# enter the source file here
remote_source_file = '/data/blobs.tif'
# enter the destination
local_file = 'blobs.tif'
oc.get_file(remote_path=remote_source_file,
local_file=local_file)
True
Image processing#
As we downloaded the file, image processing works as usual.
image = imread(local_file)
imshow(image)
<matplotlib.image.AxesImage at 0x1d29730e760>
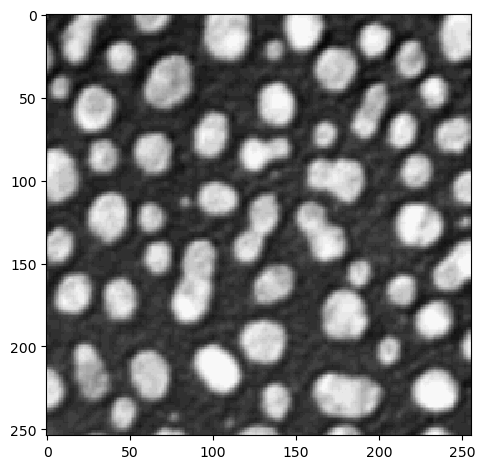
labels = voronoi_otsu_labeling(image, spot_sigma=3.5)
labels
|
nsbatwm made image
|
Uploading results#
Before uploading an image to the cloud we need to save it locally.
local_file_to_upload = "blobs_labels.tif"
imsave(local_file_to_upload, labels, check_contrast=False)
We will upload the file to this folder:
remote_folder
'/data/'
oc.put_file(remote_folder, local_file_to_upload)
True
Just to be sure, we check if the file arrived by printing out the files in the folder again.
for f in oc.list(remote_folder):
print (f.path)
/data/blobs.tif
/data/blobs_labels.tif
/data/zfish_nucl_env.tif
Logout#
When you’re done, log out!
oc.logout()
True